Python Fundamentals (Part 2.)
- Cindy Lin
- Feb 23, 2020
- 1 min read
Updated: Apr 8, 2020
Scope: Global vs. Local
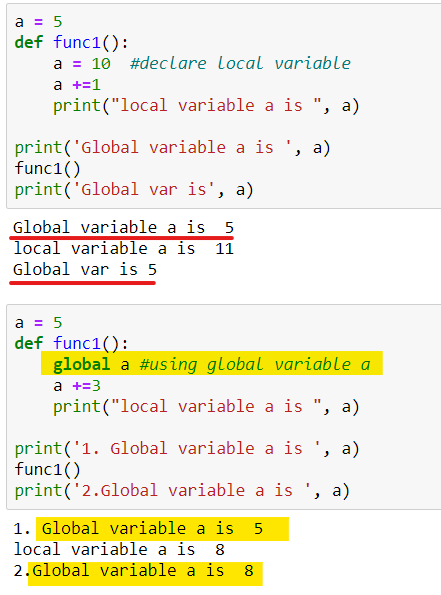
Left , Right Alignment, String Formatting
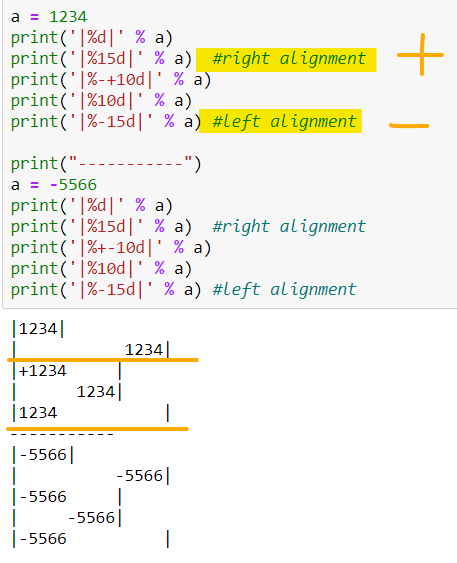
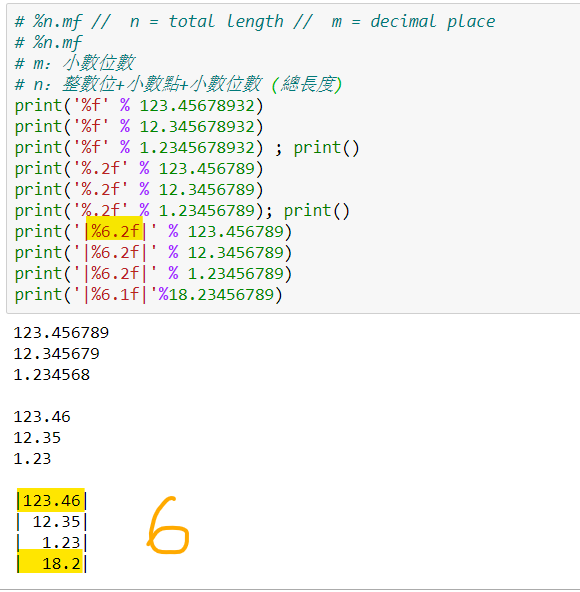
from decimal import *
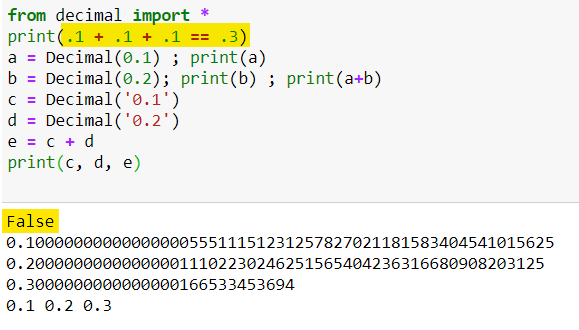
----------------------------------------------
[: : -1]
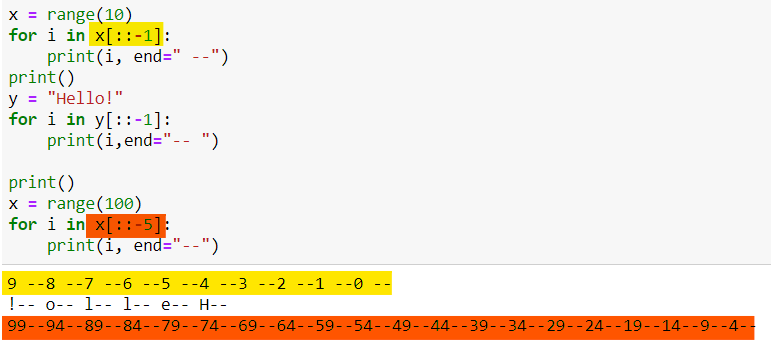
------------------------
*args , **kwargs
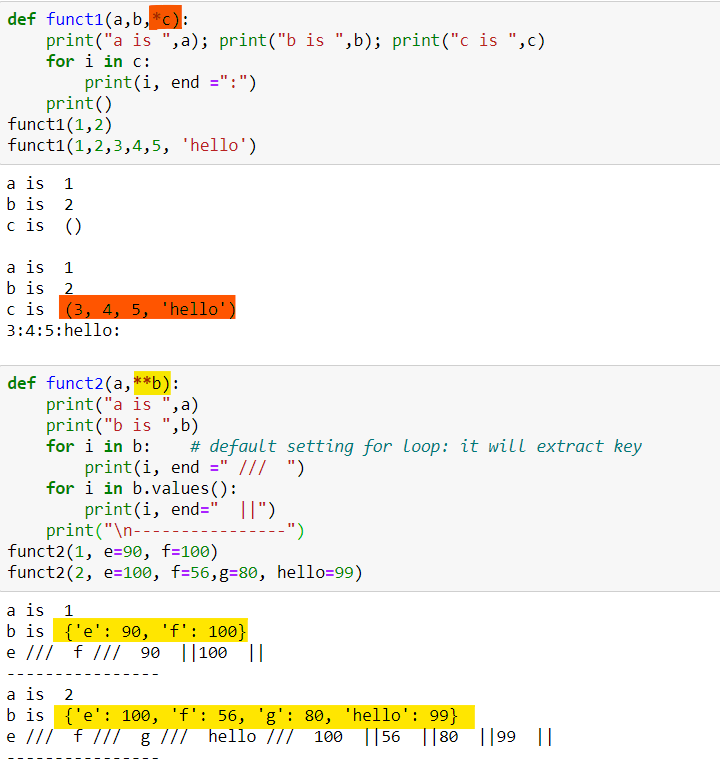
Anonymous Function:
List & Lambda
list1 = [lambda x: x**2, lambda x: x**3, lambda x:x**4]
for f in list1:
print(type(f))
print(f(3))
print('--------')
Output:
<class 'function'> 9 -------- <class 'function'> 27 -------- <class 'function'> 81 --------
Dictionary & Lambda
Example:
dict.get(key[, value])
get() Parameters
The get() method takes maximum of two parameters:
key - key to be searched in the dictionary
value (optional) - Value to be returned if the key is not found. The default value is None.

---------------------------------------------
Map & Filter
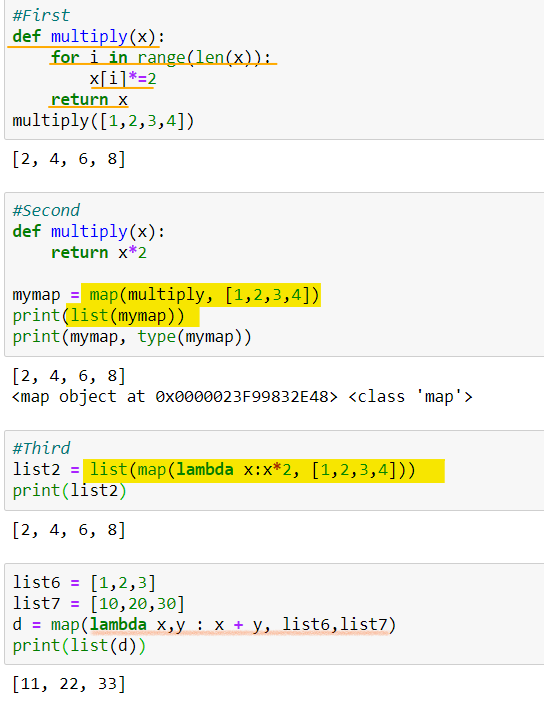

---------------------------
import random
import math
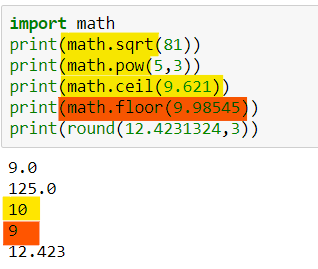
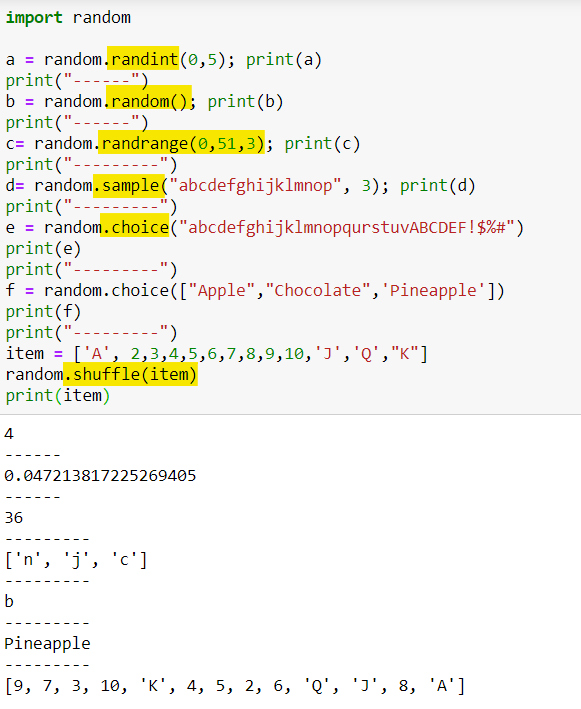
--------------------
file = open('file_name', 'w', encoding = 'utf-8')
file.write(text) # text has to be string
file.close()
----------------------
How to read file?
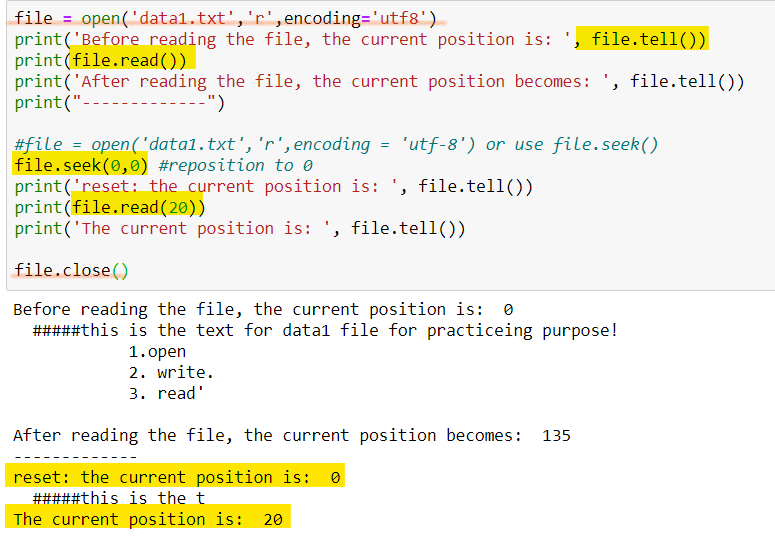
---------------------------
line.strip()
remove leading and trailing spaces
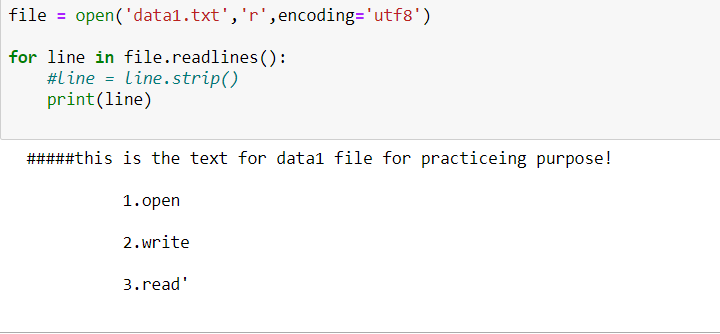
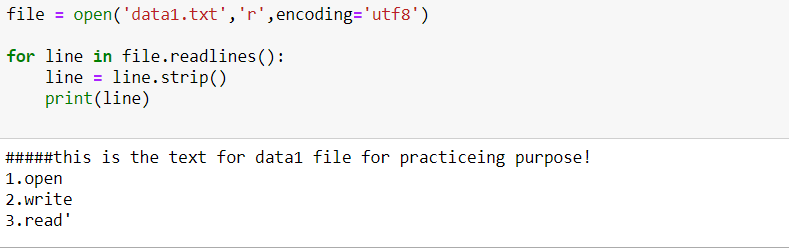
-----------------------------------
import csv
csv.reader(file) => if the data doesn't contain header/title
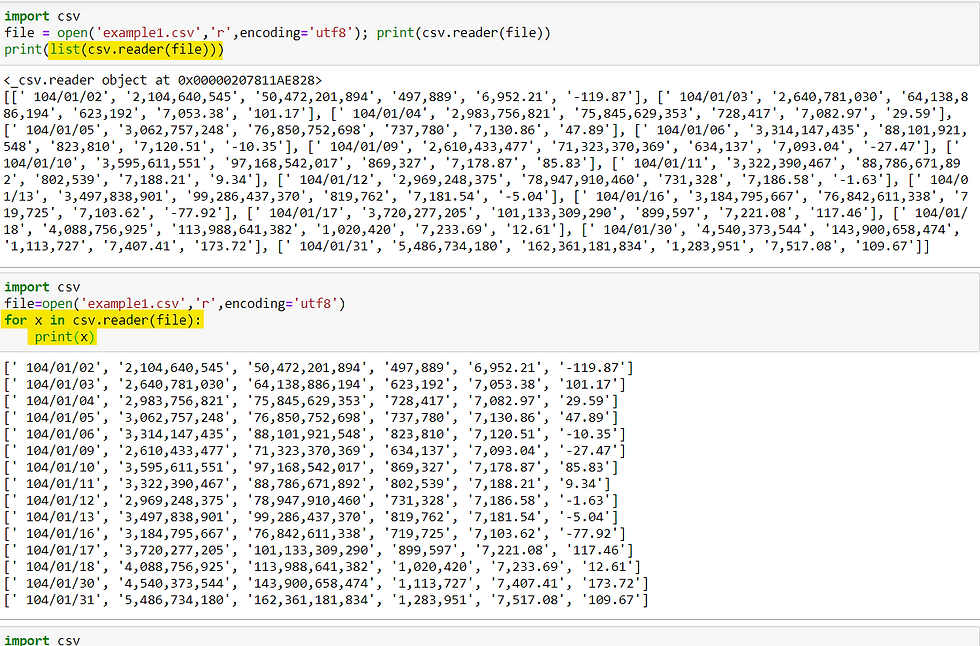
csv.DictReader(file) => if the data contain header/title
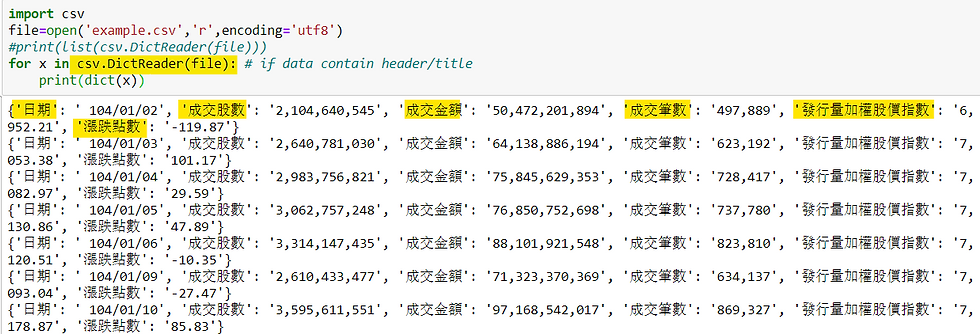
csv.writer()
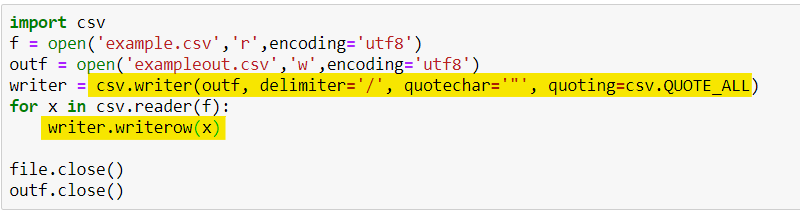
------------------------------------
json.dump() ; json.dumps() => Python to Json file
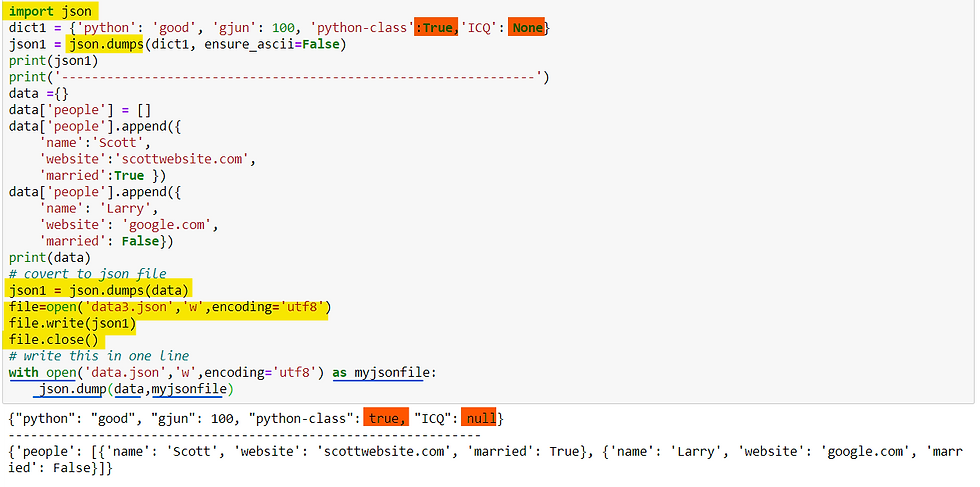
----------------------------------------------------------------------
json.load() ; json.loads() => Json to Python file

try; except
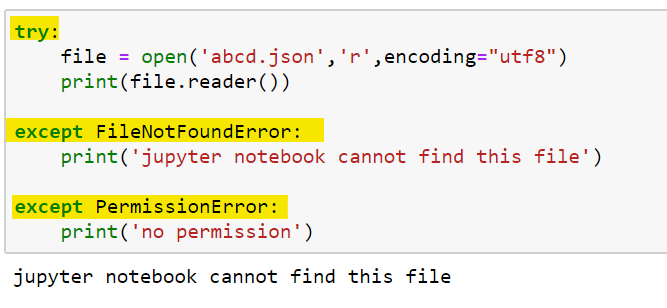
-------------------------------------------------------------------------------------
import os
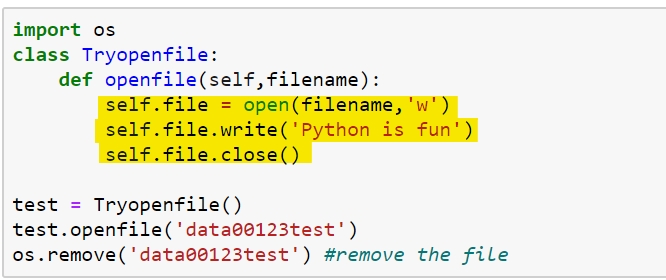
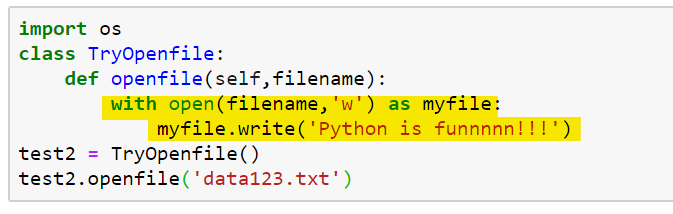
-------------------------------------
import numpy as np
np.array()
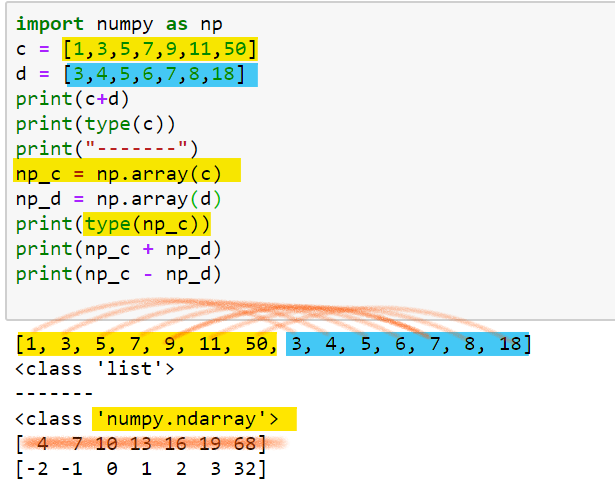
---------------------------
# ndarray.ndim
# ndarray.shape
# ndarray.size
# ndarray.dtype
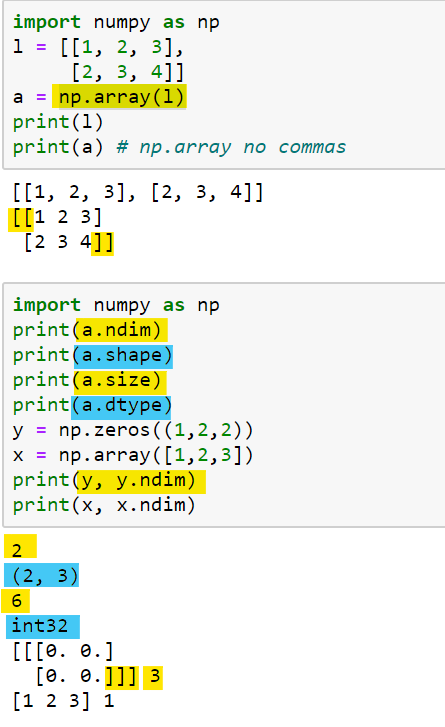
-------------------------------------------------------------------------
np.empty() ; np.ones() ; np.full() ; np.eye() ; np.diag()

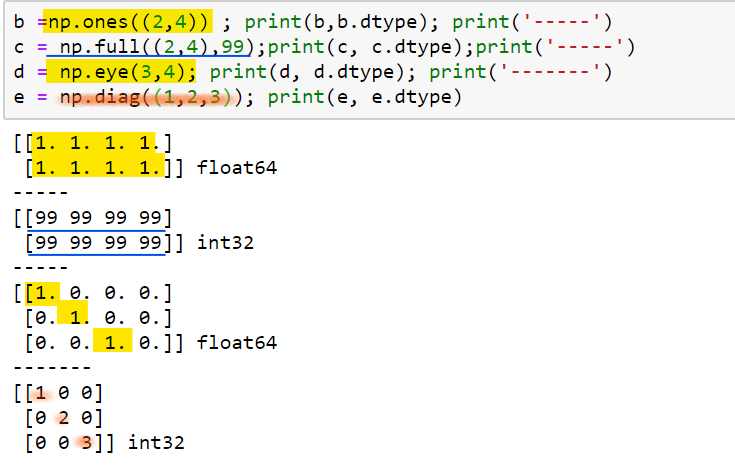
----------------------------------------------------------------------------------
np.arange() ; (decimals are allowed )
np.arange().reshape()
np.linspace(); (default number is 50, default endpoint will be included)

--------------------------------------------------------------
How to filter np.array() ?
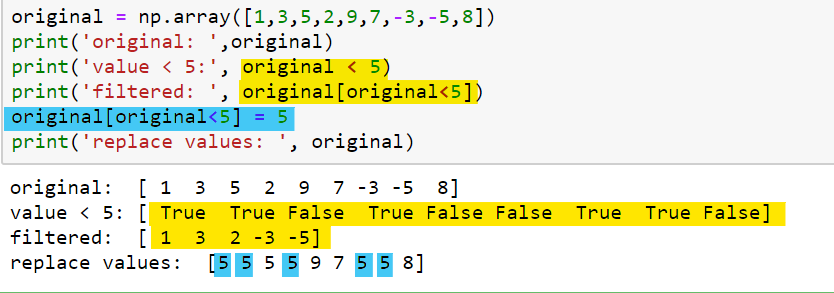
-------------------------------------------------------------
Extract values, update values from array

Extract values with list, tuple
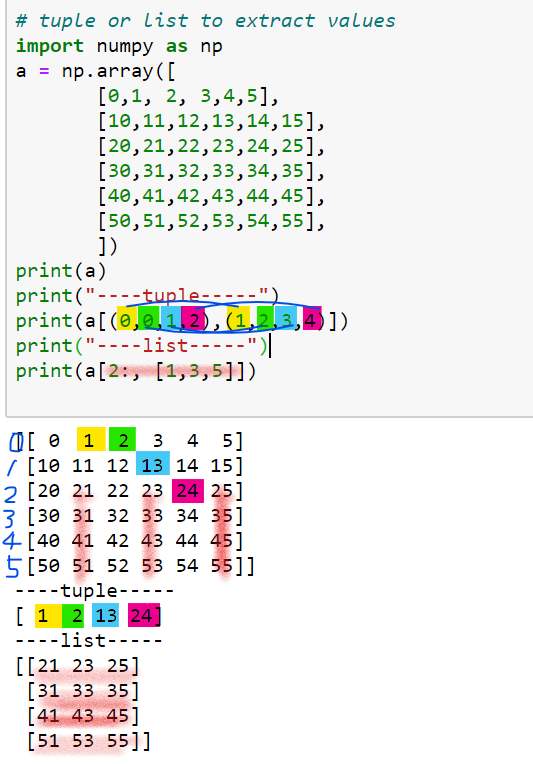
---------------------------------------------------------------------
Difference between resize and reshape
resize: creates new array
reshape: modifies the original array
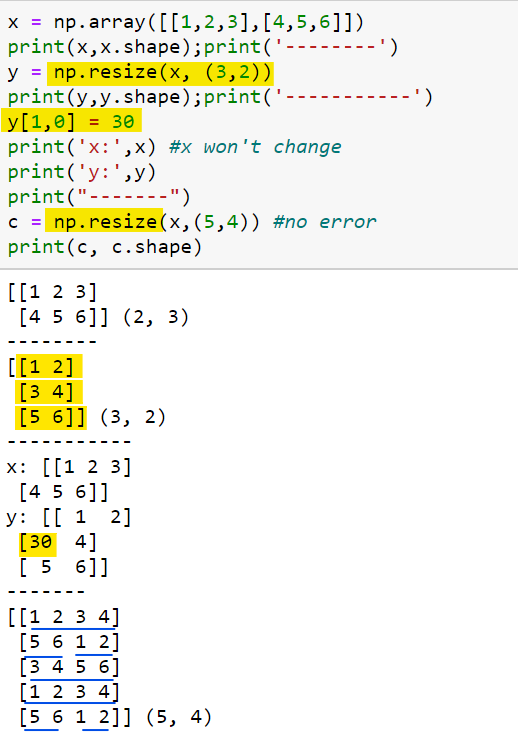

-------------------------------------------------------------------------------------
np.reciprocal() ; np.round(value, decimal = n)
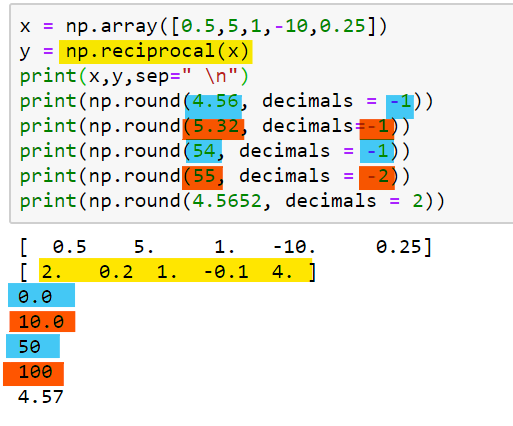
Comments